OBSTACLE AVOIDING ROBOT
What are we going to build?
We will build a simple obstacle avoiding robot that will avoid obstacles which are in its path.
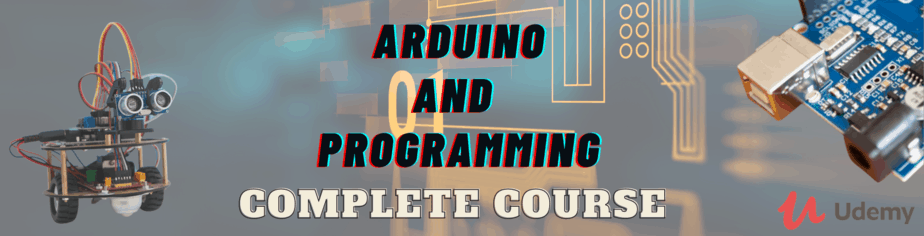
There can be two possible robots that can be built:-
1. A simple robot with no rotation of the distance sensor.
2. A mediocre robot with the rotation of the distance sensor.
Let’s gather the components needed:-
1. Arduino mega. (or any other Arduino i.e Arduino Nano or Arduino UNO).
2. L298N Dual H Bridge Stepper Motor Driver.
3. DC hobby BO motors.
4)HC-SR04 Ultrasonic Distance Measuring Sensor Module
5)9v battery
6)Robot chassis
Obstacle avoiding robot Circuit Diagram.
Connections of Ultrasonic sensor –
1. VCC – VCC terminal of Arduino.
2. GND – GND terminal of Arduino.
3. Trigpin – digital pin 9 on Arduino.
4. Echo pin – digital pin 10 on Arduino.
Connections of L298N –
1. +12V – Positive terminal of the battery.
2. GND – a)GND of Arduino b)Negative terminal of the battery.
3. Input terminal 1 – Pin 4
4. Input terminal 2 – Pin 5
5. Input terminal 3 – Pin 6
6. Input terminal 4 – Pin 7
7. The output terminal 1 – Positive of the first motor.
8. The output terminal 2 – Negative of the first motor.
9. The output terminal 3 – Positive of the second motor.
10. The output terminal 4 – Negative of the second motor.
Code
int trigPin = 9;
int echoPin = 10;
int revright = 4; //REVerse motion of Right motor
int fwdleft = 7;
int revleft= 6;
int fwdright= 5; //ForWarD motion of Right motor
int c = 0;
void setup() {
//Serial.begin(9600);
pinMode(5, OUTPUT);
pinMode(6, OUTPUT);
pinMode(4, OUTPUT);
pinMode(7, OUTPUT);
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
// put your setup code here, to run once:
}
void loop() {
long duration, distance;
digitalWrite(trigPin,HIGH);
delayMicroseconds(1000);
digitalWrite(trigPin, LOW);
duration=pulseIn(echoPin, HIGH);
distance =(duration/2)/29.1;
//Serial.print(distance);
//Serial.println("CM");
delay(50);
if(distance>20)
{
forward();
}
else if(distance<20)
{
left();
delay(200);
}
}
void forward() {
digitalWrite(7, HIGH);
digitalWrite(6, LOW);
digitalWrite(5, HIGH);
digitalWrite(4, LOW);
}
void backward() {
digitalWrite(7, LOW);
digitalWrite(6, HIGH);
digitalWrite(5, LOW);
digitalWrite(4, HIGH);
}
void right() {
digitalWrite(7, LOW);
digitalWrite(6, HIGH);
digitalWrite(5, HIGH);
digitalWrite(4, LOW);
}
void left() {
digitalWrite(7, HIGH);
digitalWrite(6, LOW);
digitalWrite(5, LOW);
digitalWrite(4, HIGH);
}
void stopp() {
digitalWrite(7, LOW);
digitalWrite(6, LOW);
digitalWrite(5, LOW);
digitalWrite(4, LOW);
}
Nicely explained