Human following robot using Arduino
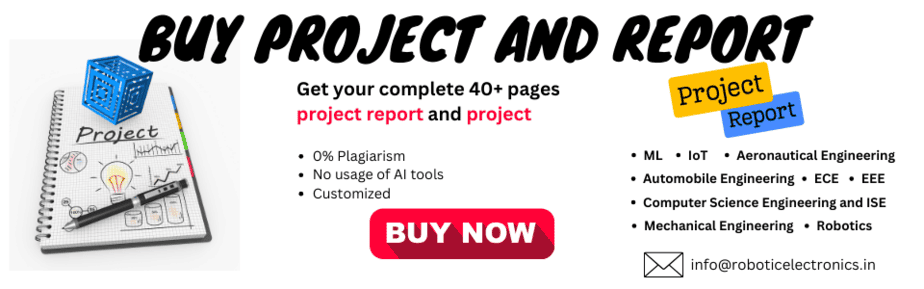
The human following robot using arduino project utilizes Arduino Uno, an ultrasonic sensor, and an L298N motor driver for obstacle detection and navigation. The robot, driven by code, halts and turns when obstacles are sensed, showcasing a basic form of autonomous behavior. This project encompasses hardware integration, software programming, and iterative testing to optimize performance. The goal is to create a versatile robot capable of dynamically navigating its environment, Let’s get started.

Components Required
These are the components required to build a Circuit diagram of Human following robot using Arduino.
• Arduino Uno R3: The main microcontroller that will process sensor data and control the motors.
• Ultrasonic sensor: This module enables wireless communication between the users’ smartphones.
• Motor driver L298N: To control the speed and direction of the motors. Popular choices include the L298N motor driver.
• DC motors (4-wheel movement): These drive the wheels and are controlled by the motor controller.
• Castor Wheel: A castor wheel is a small wheel placed at the front or back of the robot for stability. It helps the robot maintain balance and makes it easier to turn.
• Battery pack: To power up the entire controller and motor driver.
• Jumper wires: These flexible wires connect various components on the breadboard or directly on the circuit board.
• Breadboard (optional): This provides a convenient platform for prototyping and testing the circuit before final assembly.
• Construction materials (plywood, acrylic, aluminum)
Hardware Assembly for Human following robot using Arduino
• Gather Components: Make sure you have all the necessary components listed earlier.
• Mount Motors on the chassis: Attach the motors to the chassis or frame of the robot. Most
motors come with brackets that can be screwed onto the chassis. Attach wheels to the motor
shafts.
• Connect Motors to L298N Motor driver: Connect the motor terminals to the OUT1, OUT2, OUT3, and OUT4 of the L298N motor driver. Typically, motors have two wires each – connect one wire from each motor to OUT1 and OUT2, and the other two wires to OUT3 and OUT4.
• Connect L298N to Arduino: Connect the IN1, IN2, IN3, and IN4 pins of the L298N motor driver to digital pins on the Arduino (e.g., D4, D5, D6, D7). Also, connect the VCC and GND of the motor driver to a suitable power source.
• Connect Ultrasonic sensor: Connect the ultrasonic sensor to the Arduino. Connect VCC to 5V, GND to GND, Trig to a digital pin (e.g., D2), and Echo to another digital pin (e.g., D3).
• Power supply: Connect a power source (battery or power bank) to the Arduino and the motor driver. Ensure that the voltage is appropriate for both the Arduino and the motors.
• Jumper wires: Use jumper wires to make the necessary connections between components on the breadboard or directly if you are not using a breadboard.
• Mount Arduino and breadboard: Attach the Arduino and the breadboard to the robot chassis. You can use adhesive materials, brackets, or any suitable method to secure them in place.
• Final check: Double-check all connections to ensure that they are correct and secure. Make sure there are no loose wires that could cause a short circuit.

Working of Circuit diagram of Human following robot using Arduino
• Ultrasonic Sensor (HC-SR04): The ultrasonic sensor is used to measure the distance between the robot and an obstacle in front of it. The sensor sends out ultrasonic waves and calculates the time it takes for the waves to bounce back. This information is used to determine the distance to the obstacle.
• Arduino Code: The Arduino code continuously reads the distance from the ultrasonic sensor. If the measured distance is within a specified range (in this case, less than 30 cm), the code triggers the robot to stop moving. After a brief delay, the robot is programmed to turn left, the robot then resumes moving forward.
• Motor Control (L298N Motor Driver): The L298N motor driver is responsible for controlling the motors connected to the robot’s wheels. The move Forward, stop Motors, and turn Left functions in the Arduino code control the motor driver to achieve the desired movements.
• Power Supply: The power supply, typically a battery or power bank, provides the necessary power to the Arduino, motors, and motor driver.
• Behavior: In summary, the robot moves forward until it detects an obstacle. Upon detection, it tops, turns left, and then continues moving forward. This cycle repeats as the robot navigates its environment.
Circuit Diagram for Circuit diagram of Human following robot using Arduino

Pin Connection
• Connect the ultrasonic sensor VCC-Trig-Echo-GND to 5v-Digital 2-3-GND of Arduino Uno:
• Connect the L298N ENA- IN1-IN2-IN3-IN4 motor driver to Digital 8-4-5-6-7 of Arduino Uno
• Connect Motor Driver Out 1 out 2 to Gear motor 1 Gear motor 2.
• Connect Arduino uno R3 Vin to Positive (+) bread board.
• Connect Arduino uno R3 GND to negative (-) bread board.
• Connect motor driver 12v – GND to Bread board positive (+) – Negative (-).
• Connect 9v or 5v Battery to Bread board positive (+) and Negative (-) terminal.
Software Programming
• Set Up Arduino IDE, this is main software for coding the Human following robot using Arduino
• Download and install the Arduino IDE (Integrated Development Environment) from the official Arduino website: Arduino Software.
• Open the Arduino IDE and make sure your Arduino board is selected under the “Tools” menu. Choose the appropriate board type (e.g., Arduino Uno) and the port your board is connected to laptop.
• Library Inclusion: The code includes the NewPing library for working with the ultrasonic sensor.• Upload the Arduino code to the board.
Code for Circuit diagram of Human following robot using Arduino
#include
#define TRIG_PIN 2
#define ECHO_PIN 3
#define ENA 8
#define IN1 4
#define IN2 5
#define IN3 6
#define IN4 7
#define MOTOR_SPEED 150
#define TURN_DELAY 200
NewPing sonar(TRIG_PIN, ECHO_PIN);
void setup() {
pinMode(IN1, OUTPUT);
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
}
void loop() {
int distance = sonar.ping_cm();
if (distance > 0 && distance < 30) {
stopMotors();
delay(TURN_DELAY);
turnLeft();
delay(TURN_DELAY);
} else {
moveForward();
}
}
void moveForward() {
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
analogWrite(IN1, MOTOR_SPEED);
analogWrite(IN3, MOTOR_SPEED);
}
void stopMotors() {
digitalWrite(IN1, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
}
void turnLeft() {
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, HIGH);
analogWrite(IN1, MOTOR_SPEED);
analogWrite(IN3, MOTOR_SPEED);
}
Conclusion
• Power On and Obstacle Detection: Turn on the Human following robot using Arduino, test obstacle detection by placing objects in its path, and observe its stopping and turning behavior.
• Behavior Observation: Evaluate how the robot responds to obstacles, ensuring it stops, turns (as per code), and resumes forward movement.
• Parameter Fine-Tuning: Adjust distance thresholds, motor speeds, and turn delays in the code for optimal obstacle avoidance and navigation.
• Environmental and Mechanical Testing: Test the robot in different environments, checking its adaptability, and inspect mechanical components for smooth.