Introduction to numpy
Topics covered in previous Tutorials:
Crash course on python
Numpy:
It is Numerical Python.
|It is a library in python that holds linear algebra. It is a open source platform and mainly used for mathematical and logical operations in python. It has also great power to work with fourier transforms. Numpy is very much important library, that in data science we have to use this library while working with pandas and other python libraries. Basically we will be using numpy to work with 1d arrays ( vector ) and 2d arrays ( matrices ).
Why to use Numpy :
As we have already worked with lists, where we can create 1d lists and 2d lists ( matrices ) , then what would be the purpose of numpy ?
Basically numpy holds 50 times more speed than the lists. The type of the array is known as “ndarray” , which has many supporting functions to work with. Arrays are very frequently used in data science, where speed and resources are very important.
Getting started with numpy:
As we are working in jupyter notebook, It provides us the numpy library within it.
Let us import numpy library into jupyter notebook.
import numpy as np
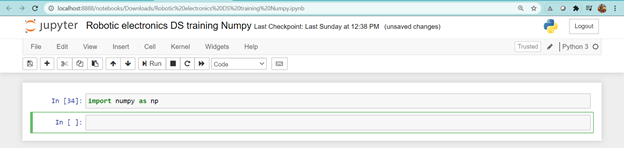
Creating arrays using numpy in python:
0D array:
Array_0 =np.array(54) # this is a zero dimensional array which consists only one element.
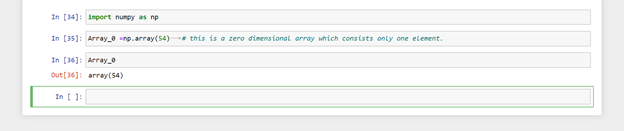
1D array:
One dimensional array means either a single row or a single column.
Array_1 = np.array( [1 ,12 ,13 ,12,43 ])
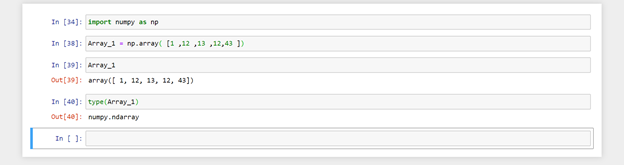
2D array:
Two dimensional array means a matrix.
Array_2 = np.array([[1, 2, 3], [4, 5, 6]])
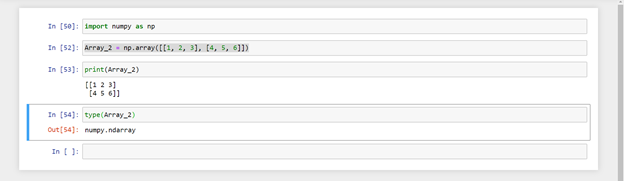
Some important functions:
- np.array( ): This function takes a sequence as a argument and converts it in to a array.
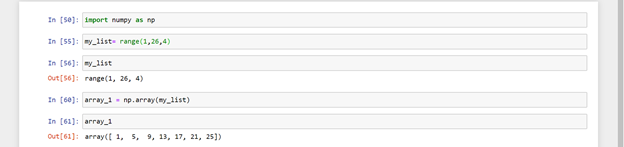
- np.arange( ): This function is similar to range( ) function.
np.arange( start, stop , difference between successive element )
If the difference parameter is empty then it automatically takes it as a unit difference.
Stop element is exclusive in the array.
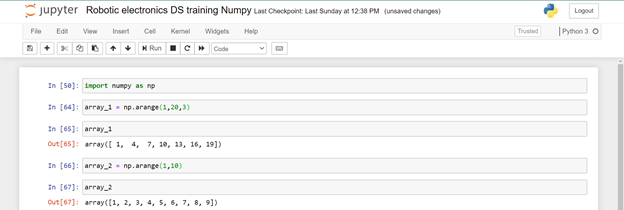
- np.zeros( ) : This function creates either a one dimensional array or two dimensional array according to the arguments given.
np.zeros( 5 ) # creates a one dimensional array of all 5 elements as zero
np.zeros(( 2,3)) # creates a two dimensional array of two rows and three columns of zero elements.
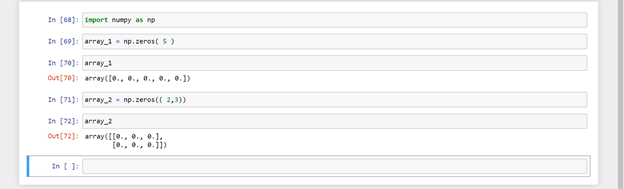
- np.ones( ) : This function is similar to zeros( ) , where this function creates one “ 1 “ as all its elements.
np.zeros( 5 ) # creates a one dimensional array of all 5 elements as ones
np.zeros(( 2,3)) # creates a two dimensional array of two rows and three columns of ones elements.
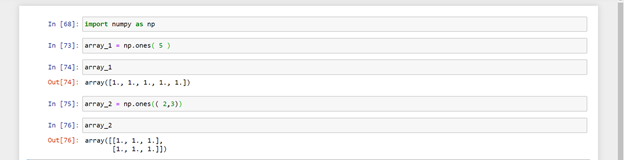
- np.linspace( ): This function is another form of creating arrays,
np.linspace( start, stop, no of elements )
Here start = starting point of the sequence
Stop = End point of sequence
no of elements = no of elements you need in the sequence , we will be getting these many number of elements equally spaced. If this argument is not mentioned it would return 50 equally spaced elements.
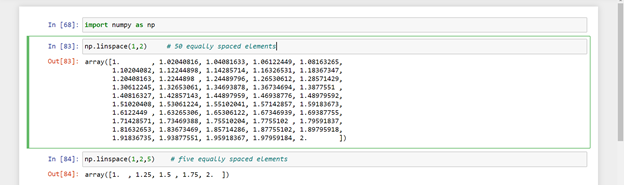
- np.eye( ): This function creates a identity matrix of given single argument.
If input is 5 , it creates a 5X5 identity matrix
identity_1 = np.eye( 5 )
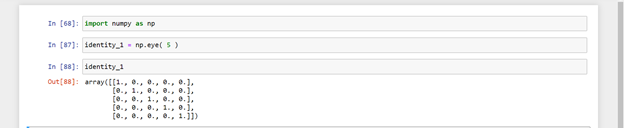
- np.random.rand( ): This function creates random elements from the size 0 to 1 and creates an array of given size.
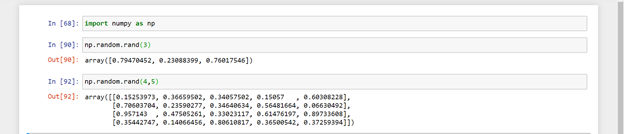
- np.random.randn( ) : This function creates random elements around the integer zero.
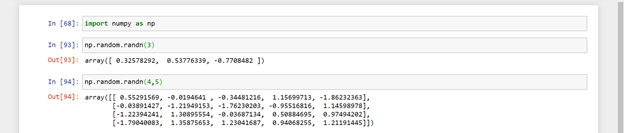
- np.random.randint( ): This is also a function to create random numbers , between the numbers ( here we are free to choose boundaries ) . This function return only integer values.
np.random.randint( lower boundary, upper boundary , size )
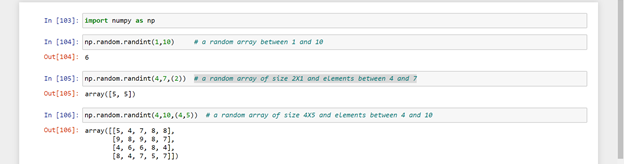
- array.reshape( ): This function reshapes the existing array according to required dimension. But the number of elements should be same or else it would give an error.
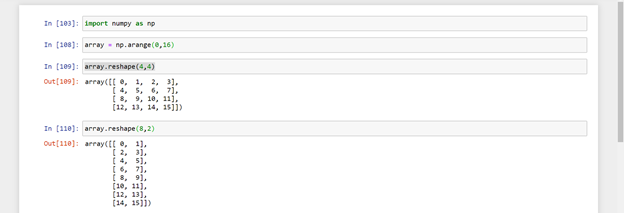
- array.max() : Returns the maximum value from the array.
- array.min() : Returns the minimum value from the array.
- array.argmin() : Return the position of the maximum value.
- array.argmin():Return the position of the minimum value.
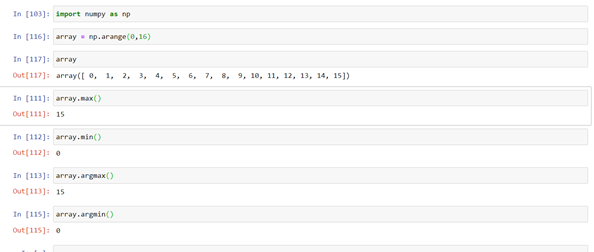
- array.shape : Returns the size of the array.
- array.dtype : Returns the data type of the elements in the array.
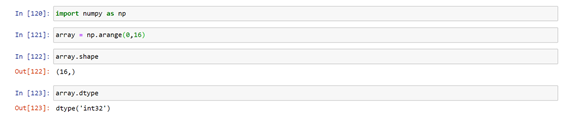
Topics will be covering in next Tutorial:
- Numpy indexing
- Slicing
- Conditional selection
- Operations in numpy
- Few universal operators