Voice controlled wheel chair using Arduino
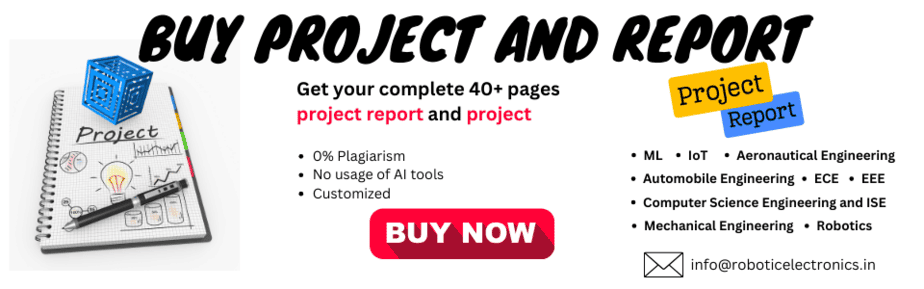
Voice controlled wheel chair using arduino is a revolutionary invention that empowers individuals with mobility challenges to control their movement using spoken commands. This project combines the power of Arduino, Bluetooth communication, and voice recognition software to create a user-friendly and accessible wheelchair experience. This guide provides a comprehensive, step-by-step approach to help you navigate the construction, programming, and fine-tuning phases of your voice-controlled wheelchair. Here’s a step-by-step guide to get you started:
Components Required

• Arduino Uno R3: The main microcontroller that will process sensor data and control the motors.
• Bluetooth module HC-05: This module enables wireless communication between the users’ smartphones.
• Motor driver L298D: To control the speed and direction of the motors. Popular choices include the L298D motor driver.
• DC motors (2 for wheelchair movement): These drive the wheels and are controlled by the motorcontroller.
• Battery pack: To power up the entire controller and motor driver.
• Wheelchair frame: This provides structural support for the entire system, including electronics and motors.
• Jumper wires: These flexible wires connect various components on the breadboard or directly on the circuit board.
• Breadboard (optional): This provides a convenient platform for prototyping and testing the circuit before final assembly.
Hardware Assembly
• Assemble the electronics: Connect the Arduino, Bluetooth module, motor driver, and DC motors according to the circuit diagram.
• Program the Arduino: Use the Arduino IDE to write code that receives Bluetooth commands, interprets them, and controls the motors accordingly.
• Install the Android App – BT Voice Control for Arduino (Google Playstore)
• Connect the battery pack: This provides power to the entire system.
• Mount the electronics: Secure the electronics on the wheelchair frame for safe and efficient operation.

Working of Voice controlled wheel chair using Arduino
• Voice Recognition: The user speaks a command into the microphone, which is converted into text by the speech recognition module.
• Bluetooth Communication: The microphone sends the audio signal to the Arduino, the text command is transmitted wirelessly via Bluetooth to the Arduino board.
• Command Processing: The Arduino uses the speech recognition library to convert the audio to text. The Arduino interprets the received command and determines the appropriate action.
• Motor Control: The Arduino translates the text to a specific motor control command based on pre-programmed commands (e.g., forward, backward, left, right, stop). The motor driver controls the DC motors to move the wheelchair according to the command.
• Adjust the speed of the wheelchair through voice commands like “faster,” “slower,” or specific speed values.
• Control the sharpness of turns by specifying the turning radius through voice commands.
Circuit Diagram of Voice controlled wheel chair using Arduino

Pin Connection
• Connect the Bluetooth module VCC-GND-TX-RX to 5V-GND-RX (Pin 10)-TX (Pin 11) of Arduino uno R3.
• Connect the motor driver VCC-GND-ENA-IN1-IN2-IN3-IN4 to Digital pins 6- 2-3-4-5 of the Arduino.
• Connect Motor Driver Out 1 out 2 to Gear motor 1 Gear motor 2.
• Connect Arduino uno R3 Vin to Positive (+) bread board.
• Connect Arduino uno R3 GND to negative (-) bread board.
• Connect motor driver 12v – GND to Bread board positive (+) – Negative (-).
• Connect 9v or 5v Battery to Bread board positive (+) and Negative (-) terminal.
Software Programming
• Set Up Arduino IDE
• Download and install the Arduino IDE (Integrated Development Environment) from the official Arduino website: Arduino Software.
• Open the Arduino IDE and make sure your Arduino board is selected under the “Tools” menu. Choose the appropriate board type (e.g., Arduino Uno) and the port your board is connected to laptop.• Install the Arduino IDE and libraries: Bluetooth library, Speech Recognition library (e.g., Julius, Sphinx)
• Upload the Arduino code to the board.
• Install the Android app on your smartphone.
• Pair the Bluetooth module with your smartphone.
• Open the app and configure the controls.
App Set-up: Download the BT Voice Control for Arduino from Google Play store.

Turn on your device Bluetooth then select Bluetooth name HC-05 after that use Voice control.

Code for Voice controlled wheel chair using Arduino
#include <SoftwareSerial.h>
SoftwareSerial BTserial(10, 11); // RX, TX pins for Bluetooth
#define ENA 6
#define IN1 2
#define IN2 3
#define IN3 4
#define IN4 5
void setup() {
Serial.begin(9600);
BTserial.begin(9600);
pinMode(ENA, OUTPUT);
pinMode(IN1, OUTPUT);
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
}
void loop() {
if (BTserial.available() > 0) {
char command = BTserial.read();
executeCommand(command);
}
}
void executeCommand(char command) {
switch(command) {
case 'F':
moveForward();
break;
case 'B':
moveBackward();
break;
case 'L':
turnLeft();
break;
case 'R':
turnRight();
break;
case 'S':
stopMoving();
break;
default:
break;
}
}
void moveForward() {
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
analogWrite(ENA, 200);
}
void moveBackward() {
digitalWrite(IN1, LOW);
digitalWrite(IN2, HIGH);
digitalWrite(IN3, LOW);
digitalWrite(IN4, HIGH);
analogWrite(ENA, 200);
}
void turnLeft() {
digitalWrite(IN1, LOW);
digitalWrite(IN2, HIGH);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
analogWrite(ENA, 200);
}
void turnRight() {
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, HIGH);
analogWrite(ENA, 200);
}
void stopMoving() {
digitalWrite(IN1, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
}
Testing and Calibration
• Test the wheelchair in a safe environment and adjust as necessary for optimal performance and user comfort.
• Choose high-quality components for reliable and safe operation.
• Follow proper circuit diagrams and safety guidelines during construction.
• Test the wheelchair thoroughly in a controlled environment before using it in public.
• Regularly maintain the wheelchair for optimal performance and safety.